Exploring the Laravel JSON API Package
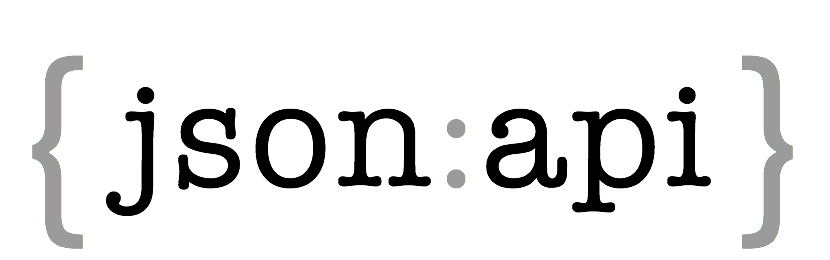
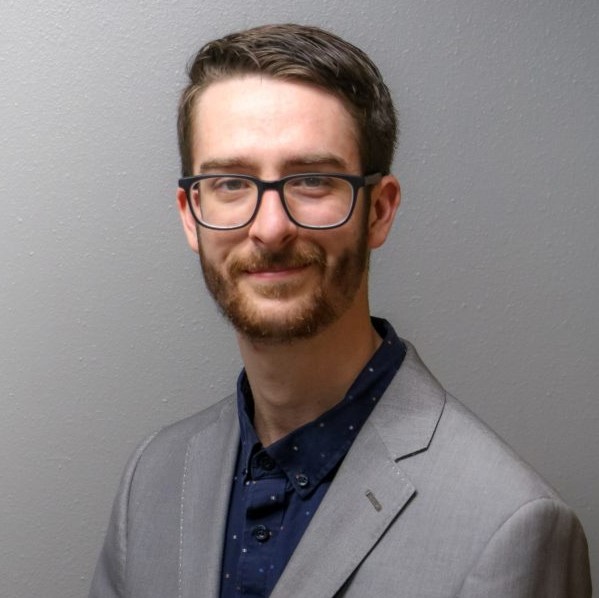
Aug 17th, 2024 by Taylor Perkins Full Stack Developer
JSON:API A Comprehensive Review
Laravel has long been known for its robust and elegant approach to building web applications. One of its many powerful tools is the Laravel JSON API package, designed to help developers work with JSON:API-compliant endpoints effortlessly. In this article, we will explore the advantages and disadvantages of the Laravel JSON API package, its ease of use for all things CRUD, and the concept of signing a contract for requests and responses.
Introduction to Laravel JSON API
The Laravel JSON API package is an official package that allows developers to build APIs that conform to the JSON:API specification. JSON:API is a standardized format for building APIs in JSON, which provides a consistent and predictable structure for both requests and responses. This standardization can reduce the friction between API consumers and providers, making integrations more predictable and easier to debug.
Ease of Use for CRUD Operations
One of the most significant advantages of the Laravel JSON API package is its ease of use for CRUD operations. The package provides a straightforward way to define resources and relationships, making it easy to create, read, update, and delete resources using standard Laravel Eloquent models.
For example, defining a resource is as simple as creating a Resource class that extends `JsonApiResource`. This class can then be used to serialize your Eloquent models into JSON:API-compliant responses. The package also handles request validation, ensuring that incoming requests adhere to the JSON:API specification.
Here’s a basic example of defining a resource:
1use LaravelJsonApi\Eloquent\Resources\JsonApiResource; 2 3class PostResource extends JsonApiResource 4{ 5 public function toArray($request) 6 { 7 return [ 8 'id' => (string) $this->getRouteKey(), 9 'type' => 'posts',10 'attributes' => [11 'title' => $this->title,12 'content' => $this->content,13 ],14 'links' => [15 'self' => route('posts.show', $this->getRouteKey()),16 ],17 ];18 }19}
The package’s ability to seamlessly integrate with Laravel’s routing, middleware, and Eloquent ORM makes it a powerful tool for developers looking to build robust and standardized APIs quickly.
Signing a Contract for Requests and Responses
One of the most compelling features of the Laravel JSON API package is its enforcement of a contract for requests and responses. This contract ensures that all interactions with your API follow a predictable structure, making it easier to manage and maintain your API over time.
In practice, this means that the package enforces strict rules on how data should be structured when it is sent to and received from your API. For example, all resource identifiers must be included in a specific format, and all errors must be structured according to the JSON:API spec. This level of standardization can reduce the likelihood of errors and improve the overall developer experience.
Advantages of Using the Laravel JSON API Package
1. Standardization and Compliance: The primary advantage of using this package is its compliance with the JSON:API specification. This ensures that your API follows a standardized format, which can simplify client-side development and reduce errors in communication between the frontend and backend.
2. Effortless CRUD Operations: Laravel JSON API provides built-in support for handling CRUD operations. This includes creating, reading, updating, and deleting resources with minimal configuration. The package integrates seamlessly with Laravel’s Eloquent ORM, allowing developers to leverage Laravel’s powerful features while adhering to JSON:API standards.
3. Flexible and Extensible: The package is highly customizable, allowing you to extend or modify its behavior to fit your specific use case. Whether you need to customize the serialization of your resources or handle complex relationships, the package provides the flexibility to do so without breaking compliance with the JSON:API standard.
4. Automated Error Handling: Error handling in APIs can be tricky, but the Laravel JSON API package offers automated error responses that conform to the JSON:API spec. This reduces the need for custom error-handling logic, making your API more robust and easier to maintain.
5. Contract for Requests and Responses: By adhering to the JSON:API specification, the package enforces a contract for requests and responses. This contract ensures that the structure of your API is predictable, making it easier for clients to interact with your API and for you to maintain consistent API behavior over time.
Disadvantages of Using the Laravel JSON API Package
1. Learning Curve: While the package aims to simplify API development, there is a learning curve associated with understanding the JSON:API specification and how to implement it correctly. Developers unfamiliar with the specification may find it challenging to get started.
2. Overhead for Simple APIs: For simple APIs that don’t require the level of standardization provided by JSON:API, the package might introduce unnecessary complexity. If your API doesn’t need to adhere strictly to the JSON:API spec, you may find that the package adds overhead without significant benefits.
3. Limited Customization for Non-Standard Use Cases: Although the package is flexible, it is designed to adhere strictly to the JSON:API standard. This can limit customization options for use cases that don’t fit neatly within the standard, requiring additional workarounds or custom implementations.
4. Performance Considerations: Depending on the complexity of your API and the number of resources being handled, the package’s strict adherence to the JSON:API spec can introduce performance bottlenecks. This is particularly relevant for APIs that need to handle large datasets or complex relationships.
Conclusion
The Laravel JSON API package offers a powerful and standardized way to build JSON:API-compliant endpoints in Laravel. While it has a learning curve and may introduce overhead for simple APIs, its advantages in terms of standardization, ease of use for CRUD operations, and contract enforcement make it a valuable tool for developers building complex, scalable APIs. By leveraging this package, you can ensure that your API is both robust and maintainable, providing a consistent experience for both developers and clients.
Whether you’re building a new API from scratch or looking to standardize an existing one, the Laravel JSON API package is worth considering for your next project.
Please sign in or create an account to join the conversation.