Laravel Dusk: A Beginner's Guide to Automated Browser Testing
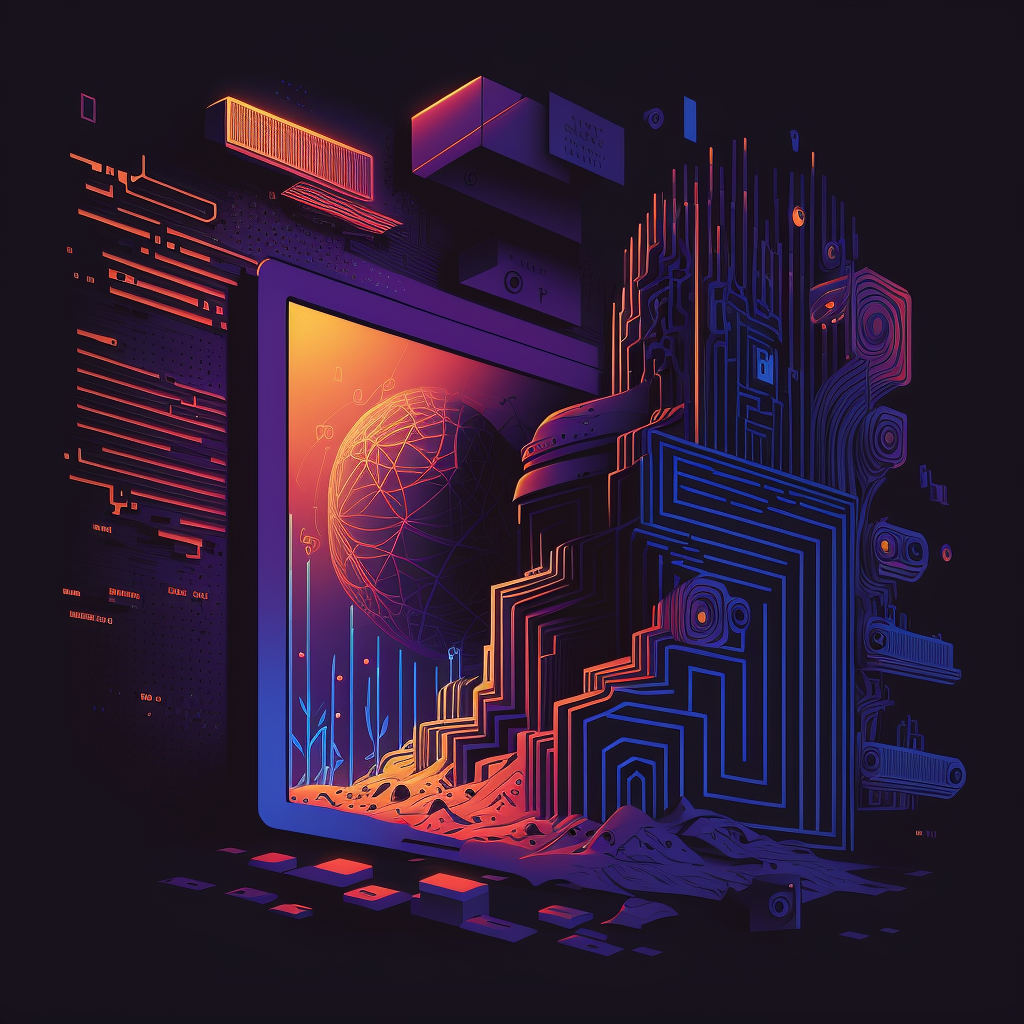
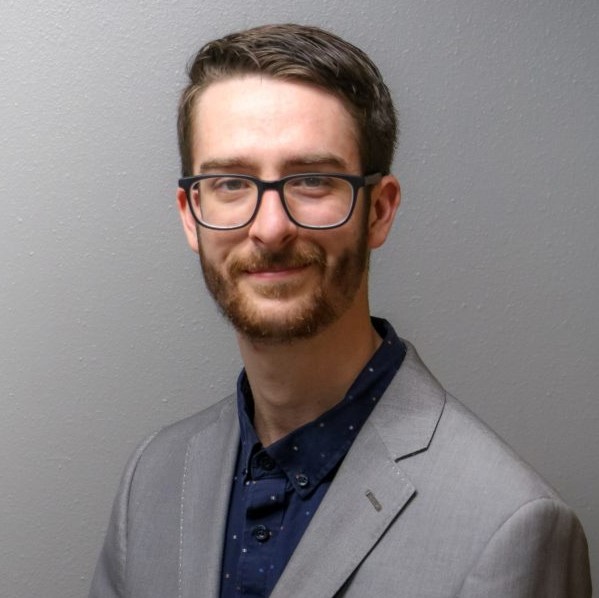
Feb 25th, 2023 By Taylor Perkins Full Stack Developer
Introduction
Laravel Dusk is a tool that allows developers to automate testing for their web applications. It is similar to unit testing, but instead of testing individual components of the application, it tests the functionality of the entire application in a real browser environment. This means that developers can test how their application looks and works from the user's perspective.
One of the key benefits of Dusk is that it allows developers to catch visual bugs. Since it tests the application in a real browser, it can detect issues such as misaligned text or broken images that traditional unit tests may miss. This can save developers a lot of time and effort by catching bugs early in the development process.
In this article, we'll cover the basics of Laravel Dusk and explore some important methods to help you write useful tests. We'll cover everything from setting up Dusk locally to writing your first browser test using Laravel Dusk.
Setting Up Laravel Dusk
Before we start exploring Laravel Dusk, we need to set it up locally. Here are the steps you need to follow:
- Install Laravel
- Install Laravel Dusk
The first step is to install Laravel on your local machine. You can do this by following the installation guide provided in the Laravel documentation. Once you have Laravel installed, the next step is to install Laravel Dusk. You can do this using Composer by running the following command in your terminal:
1composer require --dev laravel/dusk
This command will install Laravel Dusk as a development dependency in your Laravel application. After installing Laravel Dusk, the next step is to configure it. Laravel Dusk provides a command-line interface (CLI) that you can use to generate a Dusk configuration file. To generate the configuration file, run the following command in your terminal:
1php artisan dusk:install
This command will create a DuskTestCase.php file in the tests directory of your Laravel application. This file contains the necessary configuration settings for Laravel Dusk.
Writing Your First Browser Test
Now that we have Laravel Dusk set up, let's write our first browser test. In this example, we'll write a test that checks whether the login page of our Laravel application is working properly. The first step is to create a new test. You can do this by running the following command in your terminal:
1php artisan dusk:make LoginTest
This command will create a new test file called LoginTest.php in the tests/Browser directory of your Laravel application. Once you have created the test file, you can start writing your test. Here's an example of a test that checks whether the login page of your Laravel application is working properly:
1namespace Tests\Browser; 2 3use Laravel\Dusk\Browser; 4use Tests\DuskTestCase; 5 6class LoginTest extends DuskTestCase 7{ 8 public function testLogin() 9 {10 $this->browse(function (Browser $browser) {11 $browser->visit('/login')12 ->assertSee('Login')13 ->type('email', 'john@example.com')14 ->type('password', 'password')15 ->press('Login')16 ->assertPathIs('/home');17 });18 }19}
In this example, we are using the browse() method to interact with the browser. We first visit the login page, then we assert that the page contains the word "Login". Next, we fill in the email and password fields with the values "john@example.com" and "password" respectively, and then we press the login button. Finally, we assert that we have been redirected to the /home page. Once you have written the test, you can run it using the following command:
1php artisan dusk
This command will start the Laravel Dusk test runner and run all of the browser tests in your Laravel application. If everything is working properly, you should see a green message indicating that the test passed successfully.
Tips and Tricks for Using Laravel Dusk
Now that we have covered the basics of Laravel Dusk, let's explore some tips and tricks that will help you make the most of this powerful tool.
Use the artisan dusk Command
The artisan dusk command is the easiest way to run your browser tests in Laravel Dusk. This command will start the Laravel Dusk test runner and run all of the browser tests in your Laravel application.
Use the waitFor Method
The waitFor method is a powerful method that you can use to wait for certain elements to appear on the page before continuing with your test. For example, if you want to wait for a button with the ID submit to appear on the page, you can use the following code:
1$browser->waitFor('#submit');
Use the assertVisible and assertNotVisible Methods
The assertVisible and assertNotVisible methods are useful for checking whether certain elements are visible on the page. For example, if you want to check whether a button with the ID submit is visible on the page, you can use the following code:
1$browser->assertVisible('#submit');
Similarly, if you want to check whether a button with the ID submit is not visible on the page, you can use the following code:
1$browser->assertNotVisible('#submit');
Use the storeScreenshot Method
The storeScreenshot method is a useful method that you can use to take a screenshot of the current page and store it for later use. For example, if you want to take a screenshot of the current page and store it in a directory called screenshots, you can use the following code:
1$browser->storeScreenshot('screenshots/screenshot.png');
Use the visitRoute Method
The visitRoute method is a useful method that you can use to visit a specific route in your Laravel application. For example, if you want to visit the route user.show with the ID 1, you can use the following code:
1$browser->visitRoute('user.show', 1);
Use the value Method
The value method is a useful method that you can use to retrieve the value of an input field. For example, if you want to retrieve the value of an input field with the ID email, you can use the following code:
1$value = $browser->value('#email');
Use the assertSee and assertDontSee Methods
The assertSee and assertDontSee methods are useful for checking whether certain text is present on the page or not. For example, if you want to check whether the text "Hello World" is present on the page, you can use the following code:
1$browser->assertSee('Hello World');
Similarly, if you want to check whether the text "Goodbye World" is not present on the page, you can use the following code:
1$browser->assertDontSee('Goodbye World');
Use the select and radio Methods
The select and radio methods are useful for selecting options from a select box or radio button group. For example, if you want to select the option with the value "1" from a select box with the ID my-select, you can use the following code:
1$browser->select('my-select', '1');
Similarly, if you want to select a radio button with the value "2" from a group of radio buttons with the name my-radio, you can use the following code:
1$browser->radio('my-radio', '2');
Use the waitForText Method
The waitForText method is a useful method that you can use to wait for certain text to appear on the page before continuing with your test. For example, if you want to wait for the text "Welcome to my page" to appear on the page, you can use the following code:
1$browser->waitForText('Welcome to my page');
Use the with Method
The with method is a useful method that you can use to chain multiple actions together. For example, if you want to visit a page, fill out a form, and submit it all in one go, you can use the following code:
1$browser->visit('/form')2 ->with('form', [3 'name' => 'John Doe',4 'email' => 'john@example.com',5 ])6 ->press('Submit');
In this code, we first visit the /form page, then we use the with method to fill out the form with the given data, and finally we submit the form by pressing the submit button.
Use the keys Method
The keys method is a useful method that you can use to simulate keyboard actions, such as typing, pressing keys, or releasing keys. For example, if you want to type the text "Hello World" into an input field, you can use the following code:
1$browser->keys('#my-input', 'Hello World');
Similarly, if you want to simulate pressing the enter key, you can use the following code:
1$browser->keys('#my-input', '{enter}');
Use the resize Method
The resize method is a useful method that you can use to resize the browser window to a certain size. For example, if you want to resize the browser window to a width of 800 pixels and a height of 600 pixels, you can use the following code:
1$browser->resize(800, 600);
Use the pause Method
The pause method is a useful method that you can use to pause the test for a certain amount of time, in milliseconds. For example, if you want to pause the test for 1 second, you can use the following code:
1$browser->pause(1000);
Conclusion
In conclusion, Laravel Dusk is a powerful and flexible tool that allows you to easily write comprehensive browser tests for your Laravel applications. It provides an expressive and easy-to-use API for interacting with your application's frontend, allowing you to write browser tests for your application with ease. By leveraging the power of Selenium and the simplicity of Laravel, you can write tests that are easy to read, maintain, and execute. In this article, we have covered the basic concepts of Laravel Dusk, including setting up the environment, visiting pages, interacting with elements, and making assertions.
I hope you found this article useful. If you have any questions or comments, please feel free to leave them below. Thank you for reading!
Please sign in or create an account to join the conversation.